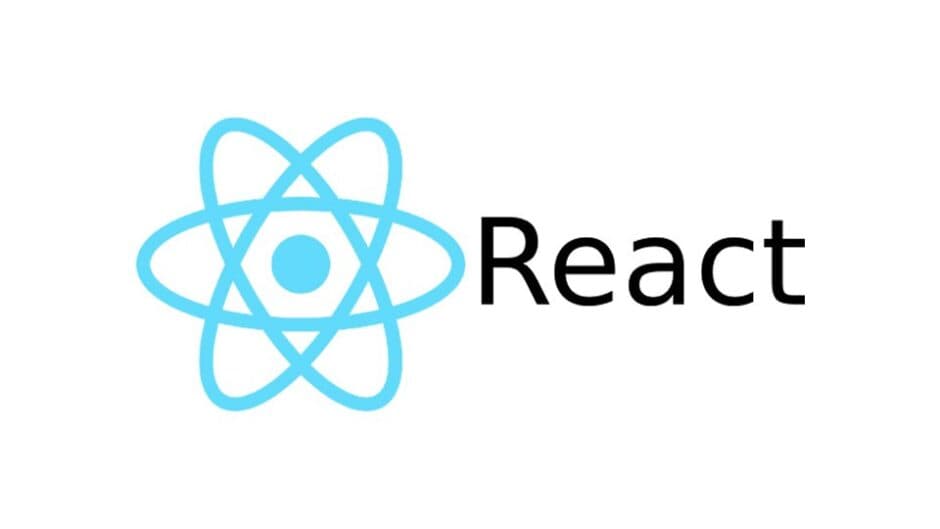
React事始め
Reactでの開発
Reactで本格的な開発を行う
1. Viteでプロジェクトを作成する
まず、Viteを使ってReactプロジェクトを作成します。ターミナルで以下のコマンドを実行してください。
npm create vite@latest
そのあと、いつもの通りプロジェクト名(仮にmy-react-appとします)、React,JavaScriptと指定していきます。
2. プロジェクトのセットアップ
プロジェクトディレクトリに移動して、依存関係をインストールします。
cd my-react-app npm install
3. Material UIのインストール
Material UIとその依存関係をインストールします。
npm install @mui/material @emotion/react @emotion/styled npm install @mui/material @mui/styled-engine-sc styled-components
4. main.jsxの変更
教科書に合わせてAppのimportを変更します。 ついでに不要なindex.cssのimportを削除します
import { StrictMode } from 'react' import { createRoot } from 'react-dom/client' // import './index.css' import { App } from './App' createRoot(document.getElementById('root')).render( <StrictMode> <App /> </StrictMode>, )
5. Material UIコンポーネントの使用
src/App.jsxに移動し、Chakra UIのコンポーネントを試してみましょう。以下は、ボタンコンポーネントを追加した例です:
import Button from '@mui/material/Button'; export const App = () => { return ( <div> <Button variant="contained" color="primary"> クリックしてね </Button> </div> ); }
6. 開発サーバーの起動
開発サーバーを起動してアプリを確認します。
npm run dev
天気予報アプリの開発
このサンプルを使い、天気予報APIから取得したデータを表示するアプリを開発します。
APIからデータを取得する際は標準のfetchでもよいですが、axiosを使う方が便利のためインストールします。
npm install axios
APIの仕様は下記
気象庁の天気予報JSONファイルをWebAPI的に利用したサンプルアプリ
import { useState, useEffect } from 'react'; import axios from 'axios'; import { Container, Typography, Button, CircularProgress, Card, CardContent } from '@mui/material'; export const App = () => { const [weather, setWeather] = useState(null); const [loading, setLoading] = useState(false); const [error, setError] = useState(null); useEffect(() => { fetchWeather(); }, []); const fetchWeather = async () => { setLoading(true); setError(null); try { // 130000は東京のエリアコード const url = "https://www.jma.go.jp/bosai/forecast/data/overview_forecast/130000.json" const response = await axios.get(url); // dataの収納 weatherにデータが入る console.log(response.data) setWeather(response.data); console.log(weather) } catch (err) { setError('データの取得に失敗しました。'); } finally { setLoading(false); } }; const handleSearch = () => { fetchWeather(); }; return ( <Container maxWidth="sm" style={{ marginTop: '2rem' }}> <Typography variant="h4" gutterBottom>天気予報アプリ</Typography> <Button variant="contained" color="primary" onClick={handleSearch}> 検索 </Button> {loading && <CircularProgress style={{ marginTop: '1rem' }} />} {error && <Typography color="error" style={{ marginTop: '1rem' }}>{error}</Typography>} {weather && ( <Card style={{ marginTop: '2rem' }}> <CardContent> <Typography variant="h5">{weather.targetArea}</Typography> <Typography variant="body1">今日の天気: {weather.text}</Typography> </CardContent> </Card> )} </Container> ); }