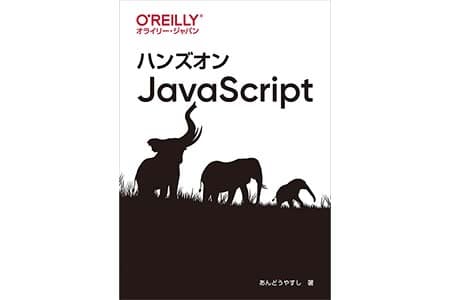
【ハンズオンJavaScript】最速でJavaScriptを扱えるようになる 3日目 クイズゲーム③
JavaScriptでクイズゲームを作る③
ハンズオンJavaScriptを進めていく上での補助記事その3です。
- やること:配列(array),for文, for-of文, if文
- やらないこと:Object,アロー関数 など
クイズゲームの作成 その3
配列とfor文を使い、処理を繰り返す。 (P75 2.12 配列 P104 3.5処理を繰り返す)
さらに短くしていきます。今回作った関数removeAnswerを3回繰り返しているので、これを配列(array) とfor文 を使って短縮します。
まずは配列(array) についてです。
配列を使うことで、複数の要素(リテラル)を順番にまとめて管理することができます。 宣言の仕方は[ ]で囲むことです。
fruits = ["りんご","みかん","バナナ"]
次に繰り返し処理を行うfor文です。 これは他の言語とほぼ同じ書き方なので省略します。
これらを使うとremoveAnswerと書くのが1度で済みます。
<script> function removeAnswer(answer) { const answerElement = document.getElementById(answer); answerElement.removeAttribute("class"); answerElement.textContent = answer; } document.getElementById("A").addEventListener("click", function () { this.setAttribute("class", "true"); this.textContent = "正解"; buttons = ["B","C","D"]; for (let i = 0; i < 3; i++;){ removeAnswer(buttons[i]) } }) // 略 </script>
さらに、for-of文を使うとより短くなります。 Javaでいう拡張for文であり、ofの後ろに入れた配列を順番に取り出す構文です。
<script> // 略 document.getElementById("A").addEventListener("click", function () { this.setAttribute("class", "true"); this.textContent = "正解"; for (const ans of ["B","C","D"]){ removeAnswer(ans) } }) </script>
if文 (P97 3.4 処理を分岐する)
毎回["B","C","D"] や ["A","B","D"]などと特定の要素を抜く配列を書くのは面倒です。
条件分岐を行うif文を使って簡単にしたいと思います。
まずは buttons = ["A","B","C","D"]; という配列を作った上で、
if文と !== 演算子を使い、各クリックごとに特定の要素だけを除外します。
<script> // 略 buttons = ["A","B","C","D"]; document.getElementById("A").addEventListener("click", function () { this.setAttribute("class", "true"); this.textContent = "正解"; for (const button of buttons){ if (button !== "A"){ removeAnswer(button) } } }) document.getElementById("B").addEventListener("click", function () { this.setAttribute("class", "false"); this.textContent = "不正解"; for (const button of buttons){ if (button !== "B"){ removeAnswer(button) } } }) document.getElementById("C").addEventListener("click", function () { this.setAttribute("class", "false"); this.textContent = "不正解"; for (const button of buttons){ if (button !== "C"){ removeAnswer(button) } } }) document.getElementById("D").addEventListener("click", function () { this.setAttribute("class", "false"); this.textContent = "不正解"; for (const button of buttons){ if (button !== "D"){ removeAnswer(button) } } }) </script>
if文を使ってクリック動作を関数にまとめる
「このクリックをする」という処理は同じことが書かれているので、ここも一つの関数にしたいと思います。 if文使ってクリックされたボタンによって条件分岐させてあげます。
<script> // 略 buttons = ["A","B","C","D"]; function clickBotton(clickBotton) { document.getElementById(clickBotton).addEventListener("click", function () { if (clickBotton = "A"){ this.setAttribute("class", "true"); this.textContent = "正解"; } else { this.setAttribute("class", "false"); this.textContent = "不正解"; } for (const button of buttons) { if (button !== selectBotton) { removeAnswer(button) } } }) } clickBotton("A"); clickBotton("B"); clickBotton("C"); clickBotton("D"); </script>
最後の4行もforループを使ってまとめましょう。
<script> // 略 for (const btn of buttons){ clickBotton(btn) } </script>
以下、3日目の完成形です。
当初に比べてかなり短くなりました。
<script> buttons = ["A","B","C","D"]; function removeAnswer(answer) { const answerElement = document.getElementById(answer); answerElement.removeAttribute("class"); answerElement.textContent = answer; } function clickButton(clickButton) { document.getElementById(clickButton).addEventListener("click", function () { if (clickButton === "A") { this.setAttribute("class", "true"); this.textContent = "正解"; } else { this.setAttribute("class", "false"); this.textContent = "不正解"; } for (const button of buttons) { if (button !== clickButton) { removeAnswer(button) } } }) } for (const btn of buttons) { clickButton(btn) } </script>